Summary: In this programming example, we will learn to find the square root of the number in python.
There are two ways through which we can find the square root of a number:
- Using
**
operator with 0.5 as exponential value. - Using math library.
Square Root of a Number in Python using ** Operator
- Take a number as input.
- Check if the number is positive. If yes then Step 3 else Step 4.
- Find square root using
**
i.esqrt = num ** 0.5
and output the result. - End Program.
num = int(input("Enter a number \n"))
if num>0:
sqrt = num ** 0.5
print("Square root of {} is {}".format(num,sqrt))
else:
print("Invalid number")
Output:
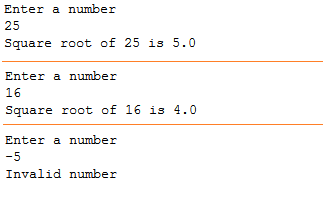
Square Root of a Number in Python using ‘math’ Library
We have to repeat almost the same steps as done in the above program. But instead of ** operator, we will use math.sqrt() method.
import math
num = int(input("Enter a number \n"))
if num>0:
sqrt = math.sqrt(num)
print("Square root of {} is {}".format(num,sqrt))
else:
print("Invalid number")
Output:
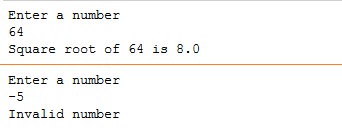
Note: Make sure to import math library to use math.sqrt() inbuilt method.